Linux Shell Scripting Beginner Tutorials – Part II
- Last Updated On: March 8, 2016
- By: Scriptcrunch Editorial
In this second series, you will learn about script debugging, conditional statements and case statements.
Debugging shell script
Everything goes wrong at some point. You can debug the shell script for error like any other programming languages using two switches “-v” and “-x”. Just append the switched before the script name to debug the script.
./ -x script.sh ./ -v script.sh
Command line arguments:
Arguments are something which will follows the command. For example , echo $0 , here echo is the command and $0 Is an argument passed to the command. $0 , by default will return the name of the shell script. Create a script file with .sh extension and try out the following example.
#! /bin/sh echo $0 $1 $2
save the file and pass any two strings with the script file.
./scriptname.sh demo string
Once you run your script with two arguments, you will get the names of two arguments and shell script name as output because $1 and $2 gets the arguments passed with the command.
Conditional Statements
You can use conditional statements like if, else in the script to make decisions. Let’s look at a simple example which uses conditional statement.
if [condition]; Then “code goes here” Elif[condition[; Then “code goes here” Else “code goes here” fi
#! /bin/sh echo "Guess this websites name" read guess if [ "$guess" = "scriptcrunch" ]; then echo "You got it right!! Bingo" else echo "Your are wrong :( try again" fi;
In the above code snippet, we get an user input from keyboard. The if statement checks if the input matches the “scriptcrunch” string. If the string is matched it will display the message from the “ then block” or else the “else” statement will be invoked and the message inside the else block will be displayed. “fi” closes the if code block. Note: When we deal with mathematical expressions, we use “–eq” instead of “=” in the if condition.
Working with loops
Shell script supports for and while loops.
Syntax: For ((initial-condition;condition;iterator)) Do command1 command2 Done
while[condition] Do Command1 Command2 Done
echo "enter you name" read name echo "how many times you want to print your name" read times echo -e "here you go n n ****************************n" for((i=1;i<=times;i++)) do echo "$name" done
In the above script, name stores the name you enter in the keyboard using read function. Times store the input value given by the user. In for loop we use times variable to print the name “n”times. ie the value given by the user.
echo -e "How many numbers you want to print???n" read number echo -e "n________________n" x=1 while [ $x -le $number ] do echo " $x" x=$(( $x + 1 )) done echo -e "n________________n"
case statements
You can use case statements in shell script. The case statement will execute the commands which match the value mentioned in the case statement. Save the following script in case.sh file and change the permissions to 755 to execute the script.
echo "enter any number between 1 and 6" read number case $number in "1") echo "you have won a BMW X6";; "2") echo "you have won a BENZ";; "3") echo "you have won a PRIUS";; "4") echo "You have won 1 million dollors";; *) echo "Sorry!! Better luck next time ";; esac
Scriptcrunch Editorial
Other Interesting Blogs
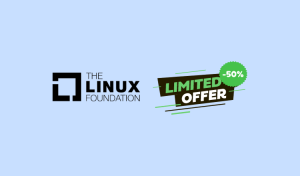
Linux Foundation Coupon for July 2024
Hi Techies, I wanted to let you know about a pretty sweet deal with the Linux Foundation Coupon that is running now.
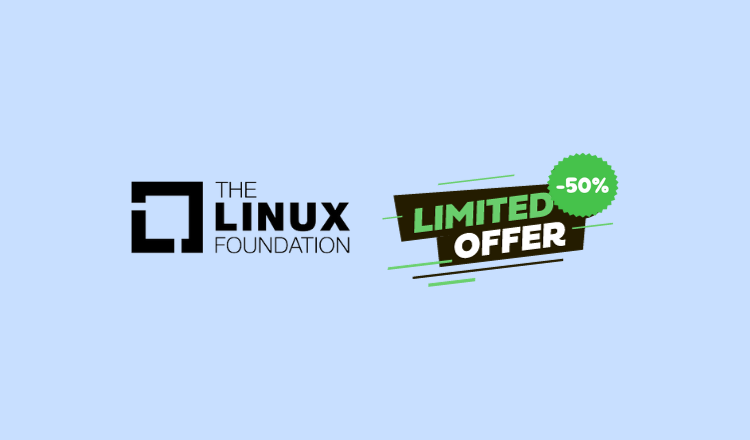
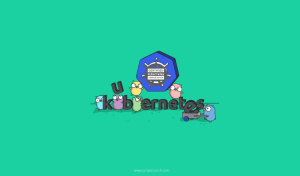
CKA Exam Study Guide: Certified Kubernetes Administrator
This comprehensive CKA certification exam study guide covers all the important aspects of the Certified Kubernetes Administrator exam and useful resources. Passing
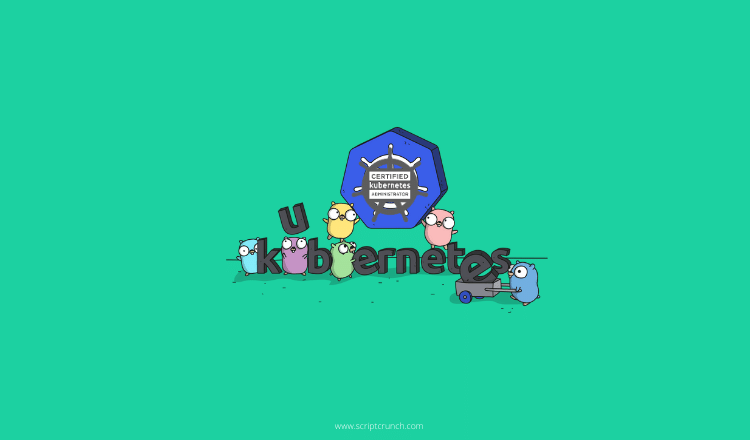
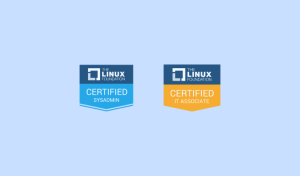
[40% OFF] Linux Foundation LFCA, LFCS & LFCT Exam Voucher Codes
Linux Foundation has announced up to a $284 discount on its Linux certification programs Linux Foundation Certified IT Associate (LFCA) and Linux
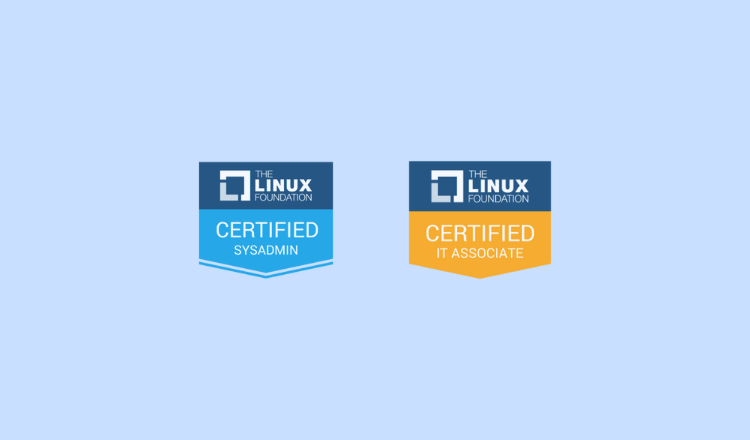