How To Process Rails Form With Model Without The Data Getting Saved To Database
- Last Updated On: February 2, 2016
- By: Scriptcrunch Editorial
Rails form_for helper used the active record class for handling the forms and saving to database. However in some cases you might not want your form data to be saved in a database. For example, if you are developing an application which creates a bucket in amazon s3, you wouldn’t want your bucket name to be stored in the database, you just pass the bucket name using form and write backend controlled codes to check if the bucket exists and if not pass the form name parameter to S3 function to create a bucket. Here we wont be needing a database to save the bucket name ,because you can use other AWS sdk functions to vie, delete and lists the buckets.
So we make little tweaks to our model class to make this work. Here I have a model named demo.rb. and it looks like this.
class Demo
include ActiveModel::Validations
include ActiveModel::Conversion
extend ActiveModel::Naming
attr_accessor :name
validates :name , presence:true
def initialize(attributes ={})
attributes.each do |name , value|
send("#{name}=" , value)
end
end
In the above code the demo class is not inheriting the active record class. Instead we are including other modules like validations, conversion and we are extending Naming. These classes have to be added for the form to work properly. For example, Active model validation lets you validate your form.
Since you are posting the data from the form using hashes you need initialize method to get those values in a hash. It loops through the attributes and uses the send method to assign the data and value.
The view has the same code as it does for the normal model. It looks like this.
<%= form_for(@demo) do |f| %>
<%= render 'shared/bucket_error_messages' %>
<%= f.label :name%>
<%= f.text_field :name%>
<%= f.submit "createbucket", class: "btn btn-default" %>
<% end %>
In controller instead of @demo.save? you should use @demo.valid? since we are not saving the data to the database. And the code looks like this
class DemosController < ApplicationController
def index
redirect_to :action => "show"
end
def new
@demo = Demo.new
end
def create
@demo = Demo.new(params[:demo])
if @Demo.valid?
<your action goes here>
end
end
The create action doesn’t save the data , instead it does the action you mention the if condition.
Scriptcrunch Editorial
Other Interesting Blogs
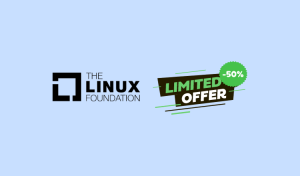
Linux Foundation Coupon for July 2024
Hi Techies, I wanted to let you know about a pretty sweet deal with the Linux Foundation Coupon that is running now.
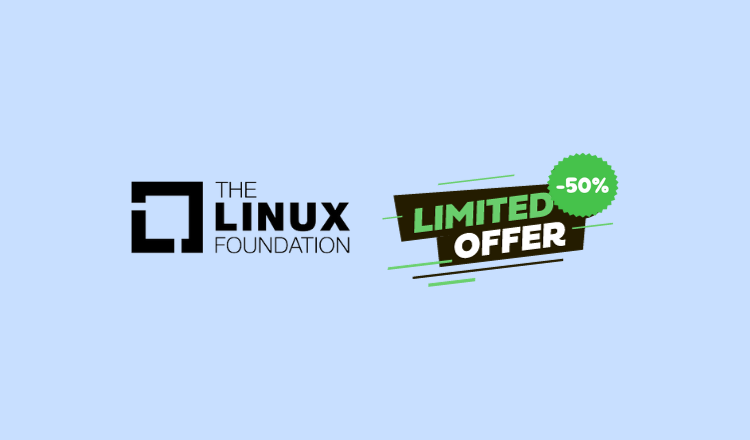
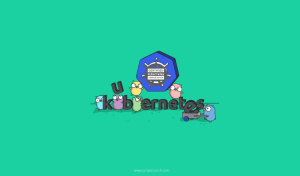
CKA Exam Study Guide: Certified Kubernetes Administrator
This comprehensive CKA certification exam study guide covers all the important aspects of the Certified Kubernetes Administrator exam and useful resources. Passing
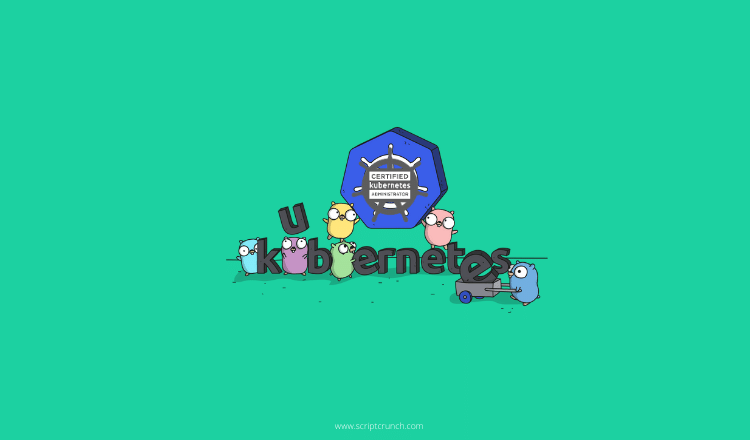
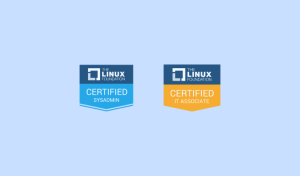
[40% OFF] Linux Foundation LFCA, LFCS & LFCT Exam Voucher Codes
Linux Foundation has announced up to a $284 discount on its Linux certification programs Linux Foundation Certified IT Associate (LFCA) and Linux
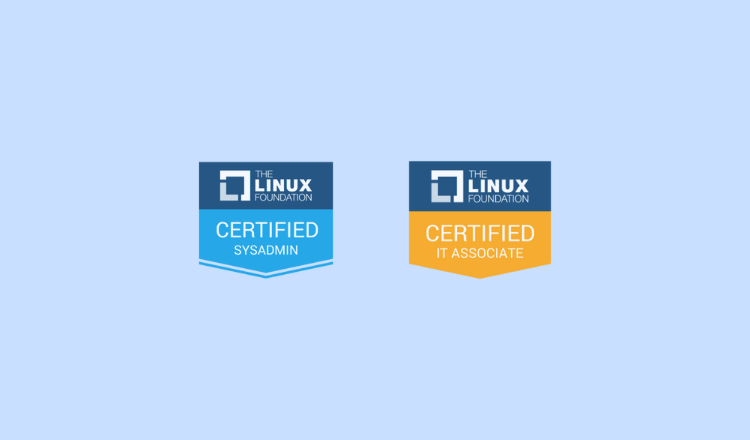