Jenkins supports many credential types based on your needs. It could be a password, secret file, SSH private key, or a token. There could be many use cases to use a groovy script to deal with credentials. A few use cases are
- You might want to create credentials in the run time.
- You might want to use a custom Groovy code with Active choice parameters.
- You might want to extend your Jenkins shared library for a credential to create functionality.
This tutorial explains how to get the stored credentials in Jenkins using a custom Groovy script and declarative pipeline script.
Script To Retrieve Jenkins Credentials
To retrieve Jenkins credentials, you should import cloudbees credentials-specific libraries. And use the lookupCredentials
function to get all the credentials stored in Jenkins.
Here is the full Groovy script to list all the Jenkins credentials.
import jenkins.*
import jenkins.model.*
import hudson.*
import hudson.model.*
def jenkinsCredentials = com.cloudbees.plugins.credentials.CredentialsProvider.lookupCredentials(
com.cloudbees.plugins.credentials.Credentials.class,
Jenkins.instance,
null,
null
);
for (creds in jenkinsCredentials) {
println(jenkinsCredentials.id)
}
You can test this script using the Jenkins script console.
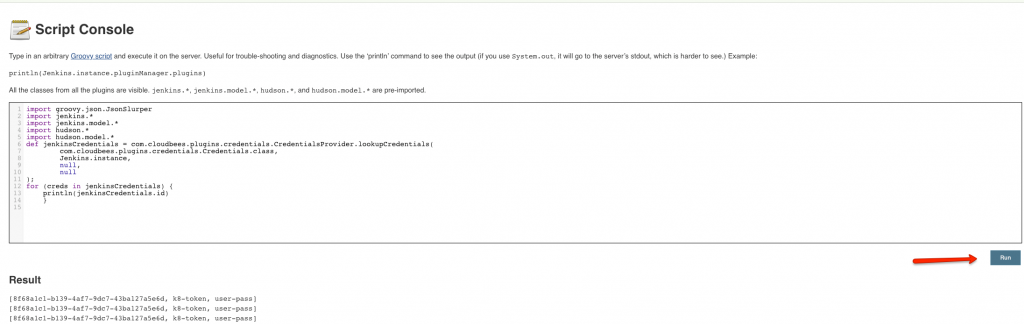
Get a Specific Stored Credential
In the above example, we have seen how to list all the Jenkins credentials.
Let’s say you have a credential of type username and password and you want to retrieve those values using the script.
For this, you need to know the ID of the credential. For example, if you have a credential with an ID named user-pass. Here is how the for loop will look like to extract the username and password.
for (creds in jenkinsCredentials) {
if(creds.id == "user-pass"){
println(creds.username)
println(creds.password)
}
}
If you run the whole script you should get the following output.
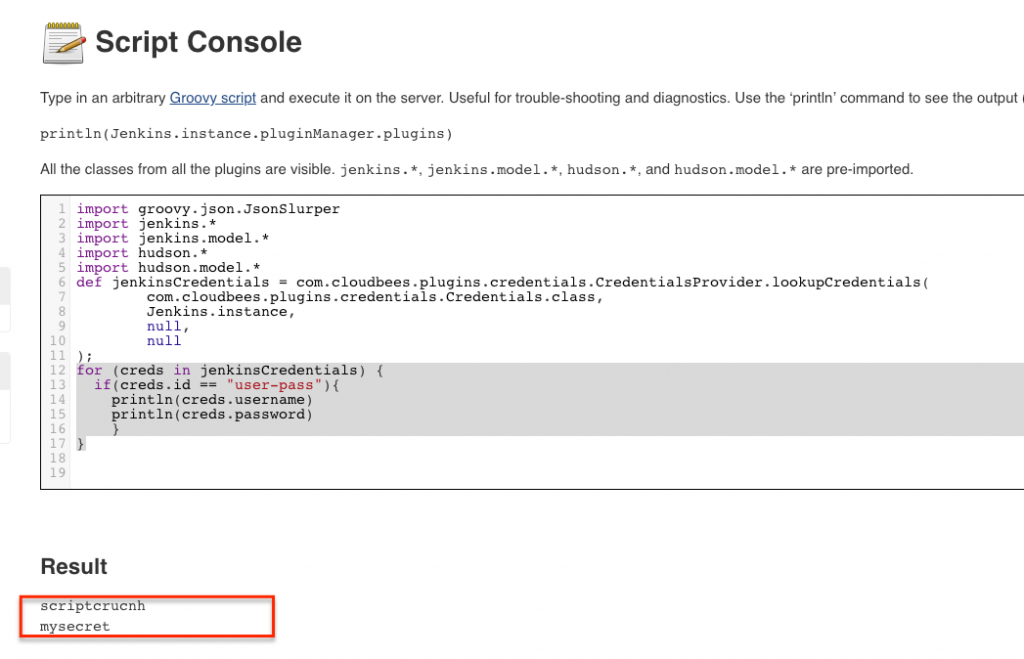
How To Find Credentials Type Variables
To retrieve the secrets, each credentials type has its own variables. It should be used in small letters.
For example, for username and password, you can use the following variables to retrieve the value.
username
password
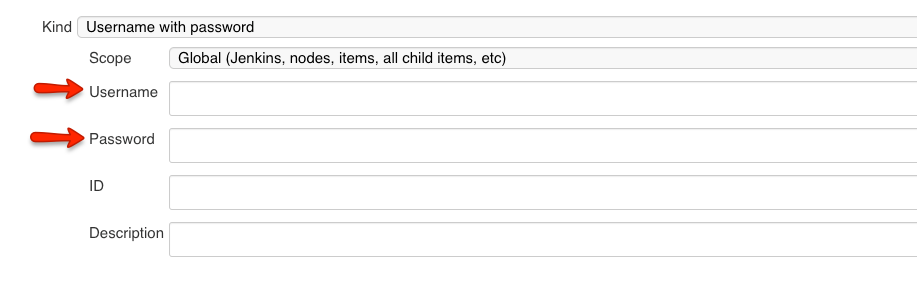
As you can see in the above image, the variables that are present during credentials creation are what we use in the code in all small letters.
Let’s say in the same way you have a private key variable as shown below. In this case, the variable name will be privateKey
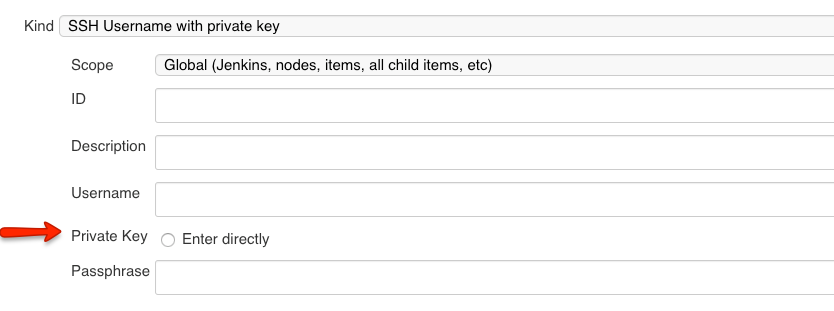
Here is the code to retrieve the SSH key from the id.
for (creds in jenkinsCredentials) {
if(creds.id == "script-ssh-creds"){
println(creds.privateKey)
}
}
Retrieve Credentials Using withcredentials jenkins
If you want to retrieve credentials using a declarative pipeline script, you can use withCredentials
function.
Here is an example of retrieving the username and password of a credential named jenkins-docker-ssh
pipeline {
agent any
stages {
stage('Hello') {
steps {
withCredentials([[$class: 'UsernamePasswordMultiBinding', credentialsId: 'jenkins-docker-ssh', usernameVariable: 'USERNAME', passwordVariable: 'PASSWORD']]) {
sh """
echo uname=$USERNAME pwd=$PASSWORD
"""
}
}
}
}
}
Conclusion
In this blog, you have learned to retrieve passwords from the Jenkins credential store.
It is not recommended to store credentials in Jenkins. Try to use secret management tools like Hashicorp vault and retrieve secrets in run time for production use cases.
Let us know in the comment section if you face any issues.
Thanks!
Base on your script I’ve created one that covers all credentials stored in my Jenkins:
I would like to redirect the output of the below command to a file, so that I can get the get the value from using shell script from that file.
println(creds.password)
Just wanted to point out that there is a small bug in the code sample for retrieving all credentials.
While looping through the ‘jenkinsCredentials’ object, your code in the loop is:
jenkinsCredentials.id
I believe it should be:
creds.id
This statement causes every id for every credential to be printed per the number of credentials in the list.